Last updated - April 13, 2023
Laravel is a popular PHP web application framework that was first introduced in 2011. It is an open-source framework that uses the model-view-controller (MVC) architectural pattern, which separates the application logic from the user interface.
Laravel development makes website and app development easier and faster. It does this by giving you a variety of features and tools that make common tasks like authentication, routing, caching, and database management easier.
One of the key advantages of Laravel is its elegant syntax, which is both expressive and easy to learn.
Laravel also includes a powerful templating engine called Blade, which enables developers to write clean and maintainable code. Laravel also has a large ecosystem of packages and extensions that make it easy for developers to add new features to their apps quickly.
Laravel is also known for its excellent documentation and active community.
The Laravel community is large and supportive and includes developers from around the world who contribute to the framework’s development and maintenance.
This has helped to make Laravel one of the most popular PHP frameworks in the world, with a large and growing number of developers using it to build web applications of all kinds.
Laravel Testing Tools and Techniques
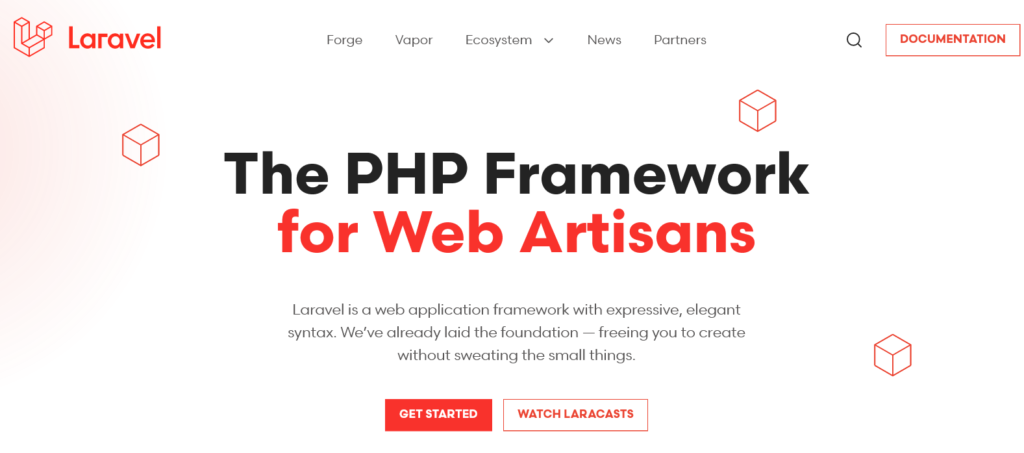
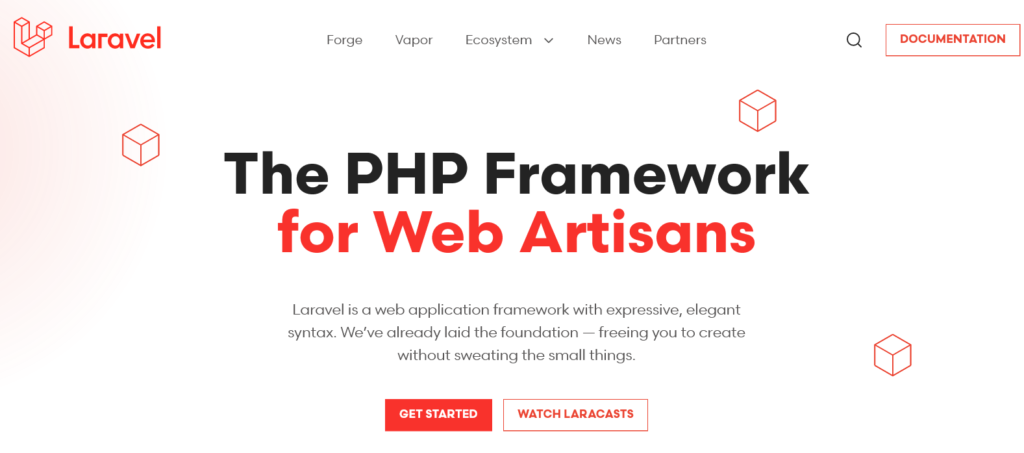
One of the key factors that contribute to Laravel’s success is its comprehensive testing tools and techniques that allow developers to build robust and reliable applications.
Testing is a critical part of software development, and Laravel provides a suite of tools and techniques that enable developers to write and run automated tests.
In this article, we’ll look at the different testing tools and methods that Laravel offers, as well as how they can be used to make applications that work better.
Unit Testing
Unit testing is an essential part of software development that enables developers to test individual components or units of code in isolation.
Laravel, being a popular PHP web application framework, provides a range of testing tools and techniques that enable developers to write and run automated tests.
One of the primary tools for unit testing in Laravel is PHPUnit, a popular unit testing framework for PHP. In this article, we will explore the basics of unit testing in Laravel using PHPUnit.
Setting Up PHPUnit in Laravel:
PHPUnit is included with Laravel by default, so there is no need to install it separately. To create a new unit test in Laravel, run the following command:
php artisan make:test TestName
This will create a new test file under the tests/Unit directory. You can then write your test cases in this file.
Writing Unit Tests:
In PHPUnit, you write test cases by defining methods in your test class. Each method represents a single test case, and it should be given a descriptive name that indicates what it is testing. PHPUnit provides a range of assertions that can be used to test various scenarios.
For example, let’s say we have a class called User that has a method called isAdmin() that returns true if the user is an administrator. We can write a PHPUnit test for this method that checks whether it returns the expected value:
<?php
use PHPUnit\Framework\TestCase;
class UserTest extends TestCase
{
public function testIsAdmin()
{
$user = new User([‘role’ => ‘admin’]);
$this->assertTrue($user->isAdmin());
}
}
In this example, we create a new instance of the User class and pass in an array that contains the user’s role. We then use the assertTrue assertion to check whether the isAdmin() method returns true.
PHPUnit provides a range of other assertions that can be used to test various scenarios.
For example, you can use the assertEquals assertion to check whether two values are equal, or the assertContains assertion to check whether an array contains a particular value.
Running Unit Tests:
To run your unit tests in Laravel, use the following command:
php artisan test
This will run all the unit tests in your tests/Unit directory. You can also run individual test files or test cases by specifying the file or method name.
Unit testing is a critical part of software development, and PHPUnit provides a powerful and flexible framework for writing and running automated tests in Laravel.
By using PHPUnit, developers can ensure that their code is reliable, robust, and secure. Whether you’re a seasoned developer or just starting out, Laravel’s testing tools and techniques can help you build better applications.
Integration Testing
Integration testing is an essential part of web application development that tests the integration of multiple components or units of code. Laravel, being a popular PHP web application framework, provides a range of testing tools and techniques that enable developers to write and run automated tests. In this article, we will explore the basics of integration testing in Laravel.
Setting Up Integration Testing in Laravel:
To set up integration testing in Laravel, you need to have a testing database and the necessary packages installed. You can create a testing database by running the following command:
php artisan make:test ExampleTest –unit
This will create a new test file under the tests/Unit directory. You can then write your integration test cases in this file.
Writing Integration Tests:
In Laravel, integration tests are written using the TestCase class provided by the framework. This class provides a range of methods that can be used to make HTTP requests and test the response.
For example, let’s say we have a route in our application that returns a JSON response containing a list of users. We can write an integration test for this route that checks whether it returns the expected response:
<?php
namespace Tests\Feature;
use Illuminate\Foundation\Testing\RefreshDatabase;
use Tests\TestCase;
class ExampleTest extends TestCase
{
use RefreshDatabase;
public function testExample()
{
$response = $this->get(‘/api/users’);
$response->assertStatus(200);
$response->assertJsonStructure([
‘*’ => [
‘id’,
‘name’,
’email’,
]
]);
}
}
In this example, we use the get method to make a GET request to the /api/users route. We then use the assertStatus method to check whether the response has a status code of 200, indicating that the request was successful. We also use the assertJsonStructure method to check whether the response contains the expected JSON structure.
Running Integration Tests:
To run your integration tests in Laravel, use the following command:
php artisan test –testsuite=Feature
This will run all the integration tests in your tests/Feature directory. You can also run individual test files or test cases by specifying the file or method name.
Integration testing is a critical part of web application development, and Laravel provides a powerful and flexible framework for writing and running automated integration tests.
By using Laravel’s testing tools and techniques, developers can ensure that their code is reliable, robust, and secure. Whether you’re a seasoned developer or just starting out, Laravel’s integration testing tools and techniques can help you build better applications.
Functional testing
Functional testing is a type of testing that checks whether the application is working as expected from the end-user’s perspective. In Laravel, functional testing is done through the use of the Laravel testing framework, which provides a suite of tools and methods for writing functional tests.
Here are the basic steps for writing and running functional tests in Laravel:
- Create a test file: To create a functional test in Laravel, you need to create a test file in the tests/Feature directory. The filename should end with Test.php, and the class name should end with Test. For example, LoginTest.php.
- Define the test methods: In the test file, you define the test methods that will be run. Each method should start with the word test and should contain the code to run the test. For example, public function testLogin().
- Make requests to the application: To simulate user interactions with the application, you can use Laravel’s actingAs() method to log in a user or get(), post(), put(), and delete() methods to make HTTP requests to your application.
- Assert the response: After making a request, you can use Laravel’s assert methods to check if the response is what you expect. For example, you can use the assertSee() method to check if a particular string is present in the response or the assertRedirect() method to check if the response redirects to the correct URL.
- Run the tests: To run the tests, you can use the php artisan test command in the terminal. This will run all the tests in the tests/feature directory.
Here’s an example of a functional test in Laravel:
<?php
namespace Tests\Feature;
use Illuminate\Foundation\Testing\RefreshDatabase;
use Illuminate\Foundation\Testing\WithFaker;
use Tests\TestCase;
class LoginTest extends TestCase
{
use RefreshDatabase;
public function testLogin()
{
$user = factory(\App\User::class)->create([
’email’ => ‘test@example.com’,
‘password’ => bcrypt(‘password’),
]);
$response = $this->post(‘/login’, [
’email’ => ‘test@example.com’,
‘password’ => ‘password’,
]);
$response->assertRedirect(‘/home’);
}
}
In this example, we’re testing the login functionality of our application. We create a user using Laravel’s factory method, make a POST request to the login route with the user’s email and password, and then assert that the response redirects to the home page.
Functional testing in Laravel is a powerful way to ensure that your application is working as expected. By following these basic steps, you can write comprehensive functional tests for your Laravel application.
Conclusion
Laravel’s testing capabilities are a crucial aspect of building robust web applications. The Laravel testing framework provides developers with a suite of tools and techniques for writing comprehensive tests that ensure the application is functioning as expected.
Functional testing is a key technique for testing web applications, and Laravel provides developers with the necessary tools and methods to write functional tests that simulate user interactions with the application.
By writing and running tests, developers can catch and fix bugs early in the development process, leading to more stable and reliable applications. Additionally, automated testing can save developers time and effort, as they can run tests automatically whenever changes are made to the codebase.
Overall, Laravel’s testing capabilities are an essential part of the framework’s appeal, and developers who take advantage of these tools and techniques can build robust, reliable applications that provide a great user experience.